Access data in a nested list
You can pull individual items in a list out into its own data object variable and get or set data. This is particularity useful to access data in a nested list.
In the following example, we will get the total cost of the Order book, for the customers and for each customer get the orders, for each order get the items in each order and then add the cost per item to a running total.
To get the total cost of the Order book (sum of the cost of all items), first you have to loop through the customers and for each customer get the orders, for each order get the items in each order and add the cost per item to a running total.
This example has three nested arrays:
-
OrderBook (object)
-
customerList []
-
currentOrders []
-
items []
-
-
-
As you loop through the customerList you need to pull out each order and map it to the currentOrders data model, then add another loop to loop through the items in the order and pull out the item into the items data model. The end result of the nested loops is to get a total for all the items.
To achieve this in TotalAgility, perform the following steps.
Create a data model
Create a data model with the following fields.
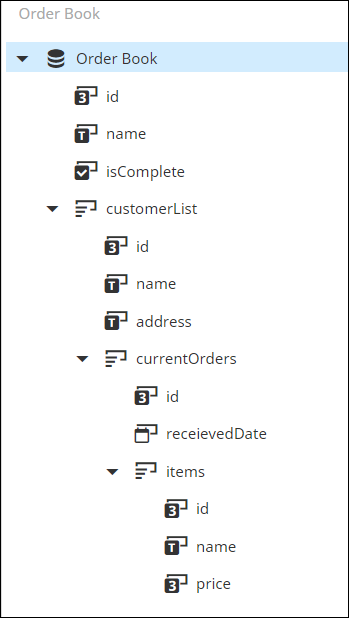
Field | Type |
---|---|
id | Number |
name | Text |
isComplete | Boolean |
customerList | List |
Fields within cutomerList | |
id | Number |
name | Text |
address | Text |
currentOrders | List |
Fields within currentOrders | |
id | Number |
receivedDate | Date |
items | List |
Fields within items | |
id | Number |
name | Text |
price | Number |
Create a process map
Create a process map called Order Book Processing.
Add variables
Add the following variables to the Order Book Processing map.
Name | Type | Value |
---|---|---|
Current Order |
Data object | currentOrders |
Current Order Found |
Bool | False |
customers |
Data object | customerList |
Customer Found |
Bool | Fasle |
Grand Total |
Decimal | 0 |
idx Current Order |
Short | 1 |
idx Customer |
Short | 1 |
idx Item |
Short | 1 |
item |
Data object | Items |
Item Found |
Bool | False |
Order Book |
Data object | Order Book |
Add nodes
Add the following nodes to the Order Book Processing map with the given configuration.

Node type | Name | Configuration |
---|---|---|
Restful service | Get Order Book |
URL parameters: 1 Verb: GET Type: JSON Response type: Variable Value: Order Book |
Loop | Each Customer |
This Loop node is for looping around Order Book.customersList. Complex variable: Order Book.customerList Current index: idx Customer Updated index: idx Customer Row found: Customer Found Return: Top level Variable members: Map the customerList column to the customer process variable. |
Decision | Customer Found? |
Condition text: Select the Customer Found process variable. Outcome > True path: Init Current Order activity |
Expression | Init Current Order |
Target variable: idx Current Order Description: Initialize index to 1 to start processing from first current order of each customer Expression: 1 |
Loop | Each Current Order |
This Loop node is for looping around OrderBook.currentOrders. Complex variable: customer.currentOrders Current index: idx Current Order Updated index: idx Current Order Row found: Current Order Found Return: Top level Variable members: Map the currentOrders column to the Current Order process variable. |
Decision | Current Order Found |
Condition text: Select the Customer Order Found process variable. Outcome > True path: Init item activity |
Expression | Init item |
Target variable: idx Item Description: Initialize index to 1 to start processing from first item of each current order of customer Expression: 1 |
Loop | Each Item |
This Loop node is for looping around items. Complex variable: Current Order.items Current index: idx Item Updated index: idx Item Row found: Item Found Return: Top level Variable members: Map the items column to the item process variable. |
Decision | Item Found? |
Condition text: Select the Item Found process variable. Outcome > True path: calculate Total activity |
Expression | Calculate Total |
When you run this expression node each time around Each item loop node, the current Order.items variable value is added to Grand Total variable to get the total item cost. Target variable: Grand Total Description: This member holds grand total of all item prices Expression: Grand Total (process variable) + item.price (process variable) |
Ordinary activity | Publish Report | Input variable: Grand Total |
End | End | Event type: Default |